作者 | Chaitanya Baweja
译者 | 火火酱,责编 | Carol
出品 | 程序人生(ID:coder_life)
Python是一种no-BS编程语言。可读性和设计简单是它广受欢迎的两个最大原因。
正如Python禅宗所说:
美丽胜于丑陋。
明了胜于晦涩。
这就是为什么我们可以通过记住一些常见的Python技巧来改进代码设计。这会为你节省很多时间,也能免去每次都要上Stack Overflow找答案的麻烦。
在你日常的编码练习中,下面的技巧会非常有用。
1.反转字符串
以下代码段使用Python切片操作来反转了一个字符串。
# Reversing a string using slicingmy_string = "ABCDE"reversed_string = my_string[::-1]print(reversed_string)# Output# EDCBA
更多信息:
https://medium.com/swlh/how-to-reverse-a-string-in-python-66fc4bbc7379
2.使用标题大小写(首字母大写)
以下代码段可用于将字母串首字母进行大写。通过使用字母串类的title()方法完成。
my_string = "my name is chaitanya baweja"# using the title() function of string classnew_string = my_string.title()print(new_string)# Output# My Name Is Chaitanya Baweja
3.在字符串中查找唯一的元素
以下代码段可被用于查找字符串中所有的唯一元素。我们可以利用“集合中的所有元素都是唯一的”这一属性。
my_string = "aavvccccddddeee"# converting the string to a settemp_set = set(my_string)# stitching set into a string using joinnew_string = ''.join(temp_set)print(new_string)
4.N次打印字符串或列表
我们可以对字符串或者列表进行乘法运算(*)。这样我们就可以将它们以任意倍数增加。
n = 3 # number of repetitionsmy_string = "abcd"my_list = [1,2,3]print(my_string*n)# abcdabcdabcdprint(my_string*n)# [1,2,3,1,2,3,1,2,3]
我们可以将这一有趣的用例用于定义一个具有常量的列表——比如说0.
n = 4my_list = [0]*n # n denotes the length of the required list# [0, 0, 0, 0]
5.列表推导式
列表推导式为我们提供了一种基于其他列表之上创建列表的好方法。
以下代码段通过将旧列表中的每个元素乘以2来创建新列表。
# Multiplying each element in a list by 2original_list = [1,2,3,4]new_list = [2*x for x in original_list]print(new_list)# [2,4,6,8]
更多信息:
https://medium.com/swlh/list-comprehensions-in-python-e8d409bb216e
6.两个变量交换值
Python使得在两个变量之间交换值(而不必使用额外变量)变得非常简单。
a = 1b = 2a, b = b, aprint(a) # 2print(b) # 1
7.将字符串拆分为子字符串列表
我们可以通过使用字符串类中的.split()方法来将字符串拆分为子字符串列表。也可以将要拆分的分隔符作为参数进行传递。
string_1 = "My name is Chaitanya Baweja"string_2 = "sample/ string 2"# default separator ' 'print(string_1.split())# ['My', 'name', 'is', 'Chaitanya', 'Baweja']# defining separator as '/'print(string_2.split('/'))# ['sample', ' string 2']
8.将字符串列表组合成为单个字符串
join()方法将作为参数传递的字符串列表组合成为单个字符串。在本例中,我们使用逗号分隔符将它们分开。
list_of_strings = ['My', 'name', 'is', 'Chaitanya', 'Baweja']# Using join with the comma separatorprint(','.join(list_of_strings))# Output# My,name,is,Chaitanya,Baweja
9.检查给定字符串是否是回文
因为我们已经讨论过如何反转字符串,所以回文就变的小菜一碟了。
my_string = "abcba"if my_string == my_string[::-1]: print("palindrome")else: print("not palindrome")# Output# palindrome
10.列表中元素的频次
有很多种方法都可以做到这一点,但我最喜欢的是用Python Counter类。
Python Counter能够追踪容器中每个元素的频率。Counter()返回字典,其中元素作为键、频率作为值。
我们还会使用most_common()函数来获取列表中most_frequent element。
# finding frequency of each element in a listfrom collections import Countermy_list = ['a','a','b','b','b','c','d','d','d','d','d']count = Counter(my_list) # defining a counter objectprint(count) # Of all elements# Counter({'d': 5, 'b': 3, 'a': 2, 'c': 1})print(count['b']) # of individual element# 3print(count.most_common(1)) # most frequent element# [('d', 5)]
11.找出两个字符串是否为字谜(Anagrams)
Counter 类的一个十分有趣的应用就是查找字谜。字谜是通过重新排列不同单词或短语的字母而形成的单词或短语。如果两个字符串的Counter 对象相等,那么它们就互为字谜。
from collections import Counterstr_1, str_2, str_3 = "acbde", "abced", "abcda"cnt_1, cnt_2, cnt_3 = Counter(str_1), Counter(str_2), Counter(str_3)if cnt_1 == cnt_2: print('1 and 2 anagram')if cnt_1 == cnt_3: print('1 and 3 anagram')
12.使用try-except-else处理错误
Python中的错误处理可以使用try/except轻松完成。向这个语句中添加一个else语句可能会十分有用,它将在try部分没有引发异常时运行。
如果你需要运行某些程序,而无需考虑异常,那么请使用finally。
a, b = 1,0try: print(a/b) # exception raised when b is 0except ZeroDivisionError: print("division by zero")else: print("no exceptions raised")finally: print("Run this always")
13.使用Enumerate来获取索键值对
以下脚本使用枚举法迭代列表中的值及其索引。
my_list = ['a', 'b', 'c', 'd', 'e']
for index, value in enumerate(my_list): print('{0}: {1}'.format(index, value))# 0: a# 1: b# 2: c# 3: d# 4: e
14.检查对象的内存使用情况
以下脚本可被用来检查对象的内存使用情况。
更多信息:
https://code.tutsplus.com/tutorials/understand-how-much-memory-your-python-objects-use--cms-25609
import sysnum = 21print(sys.getsizeof(num))# In Python 2, 24# In Python 3, 28
15.合并两个字典
在Python 2中,我们使用update()方法来合并两个字典,Python 3.5使这个过程变得更加简单。
在下面给出的这个脚本中,两个字典被合并了。当遇到交点时,将会使用第二个字典中的值。
dict_1 = {'apple': 9, 'banana': 6}dict_2 = {'banana': 4, 'orange': 8}combined_dict = {**dict_1, **dict_2}print(combined_dict)# Output# {'apple': 9, 'banana': 4, 'orange': 8}
16.执行一段代码所需的时间
以下代码段使用time库来计算执行一段代码所要花费的时间。
import timestart_time = time.time()# Code to check followsa, b = 1,2c = a+ b# Code to check endsend_time = time.time()time_taken_in_micro = (end_time- start_time)*(10**6)print(" Time taken in micro_seconds: {0} ms"). format(time_taken_in_micro)
17.展开表中表
有时,你无法确定列表的嵌套程度,而只是希望所有的元素都在一个平面列表中。
你可以这样做:
from iteration_utilities import deepflatten# if you only have one depth nested_list, use thisdef flatten(l): return [item for sublist in l for item in sublist]l = [[1,2,3],[3]]print(flatten(l))# [1, 2, 3, 3]# if you don't know how deep the list is nestedl = [[1,2,3],[4,[5],[6,7]],[8,[9,[10]]]]print(list(deepflatten(l, depth=3)))# [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
如果数组格式正确,那么Numpy flatten会是一种很好的方法。
Numpy flatten:
https://docs.scipy.org/doc/numpy/reference/generated/numpy.ndarray.flatten.htmlhttps:/docs.scipy.org/doc/numpy/reference/generated/numpy.ndarray.flatten.html
18.从列表中采样
以下的代码段使用random库从给定列表中随机生成n个样本。
import randommy_list = ['a', 'b', 'c', 'd', 'e']num_samples = 2samples = random.sample(my_list,num_samples)print(samples)# [ 'a', 'e'] this will have any 2 random values
曾有人推荐我使用Secrets库来生成用于加密的随机样本。以下代码只适用于Python 3。
import secrets # imports secure module.secure_random = secrets.SystemRandom() # creates a secure random object.my_list = ['a','b','c','d','e']num_samples = 2samples = secure_random.sample(my_list, num_samples)print(samples)# [ 'e', 'd'] this will have any 2 random values
Secrets:
https://docs.python.org/3/library/secrets.html
19.数字化
以下代码段将会把整数转换为数字列表。
num = 123456list_of_digits = list(map(int, str(num)))print(list_of_digits)# [1, 2, 3, 4, 5, 6]
20.检查唯一性
以下函数将会检查是否所有列表中的元素都唯一。
def unique(l): if len(l)==len(set(l)): print("All elements are unique") else: print("List has duplicates")unique([1,2,3,4])# All elements are uniqueunique([1,1,2,3])# List has duplicates
这些都仅仅是我在自己日常工作中发现的非常有用的小代码段,感谢你的阅读,希望能帮到你。
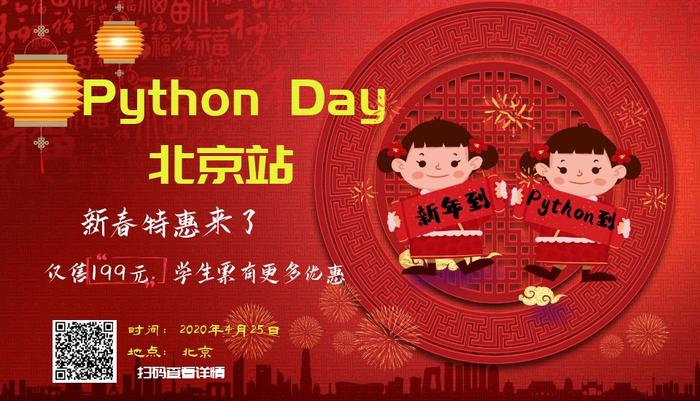
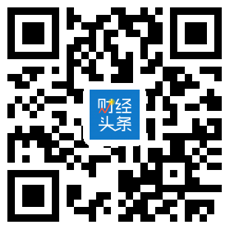
4000520066 欢迎批评指正
All Rights Reserved 新浪公司 版权所有